My use case is simple: I have a template where I’ve placed multiple text fields with unique data labels to later be populated. (I’ve also tried using pre-fill text fields) The data for it comes from my database.
I am using the nodejs sdk (i.e. docusign-esign
npm package).
I found the docs extremely confusing and I just haven’t been able to generate envelopes with the populated data from my database.
I have created this helper function to send envelopes:
import { EnvelopesApi, type EnvelopeDefinition } from 'docusign-esign';
export const sendEnvelope = async ({ envelope }: { envelope: EnvelopeDefinition }) => {
const dsApiClient = await createClient(); // createClient returns the docusign.ApiClient which I have removed in this post for maintaining simplicity
const envelopesApi = new EnvelopesApi(dsApiClient);
const { envelopeId } = await envelopesApi.createEnvelope(process.env.ACCOUNT_ID, { envelopeDefinition: envelope });
}
And now I am trying to populate these fields:
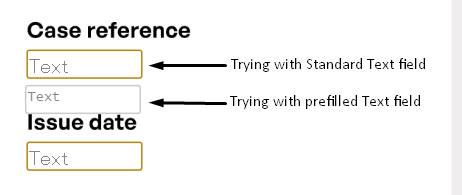
The data labels are set as so:
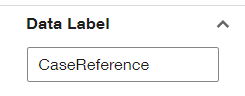
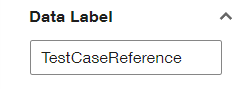
In order to fill these with data coming from my database, I am using the sendEnvelope
function like so:
// somewhere in my codebase
const project = await fetchFromMyDb();
await sendEnvelope({
envelope: {
emailSubject: 'Please review and sign this agreement',
templateId: "my-template-id",
status: 'sent',
templateRoles: e
{
email: signerInfo.email,
name: signerInfo.name,
clientUserId: signerInfo.id,
roleName: 'Client',
tabs: {
textTabs: x
{ tabLabel: 'CaseReference', pageNumber: '1', value: project.caseReference ?? '-' },
{ tabLabel: 'TestCaseReference', pageNumber: '1', value: project.caseReference ?? '-' },
],
},
},
],
},
})
This doesn’t work so I tried using composite templates like this:
// somewhere in my codebase
const project = await fetchFromMyDb();
await sendEnvelope({
envelope: {
emailSubject: 'Please review and sign this agreement',
templateId: "my-template-id",
status: 'sent',
compositeTemplates: e
{
serverTemplates: e
{
sequence: '1',
templateId: "my-template-id",
},
],
inlineTemplates: e
{
sequence: '1',
recipients: {
signers:
{
recipientId: '1',
email: signerInfo.email,
name: signerInfo.name,
clientUserId: signerInfo.id,
roleName: 'Client',
tabs: {
textTabs: t
{ tabLabel: 'CaseReference', pageNumber: '1', value: project.caseReference ?? '-' },
{ tabLabel: 'TestCaseReference', pageNumber: '1', value: project.caseReference ?? '-' },
],
},
},
],
},
},
],
},
],
},
})
Unfortunately this didn’t work either so I’m at a lost as how this is supposed to be done. Any help or even a nudge in the right direction is appreciated!